[ci] yarn format
This commit is contained in:
parent
d40edb0b67
commit
1583ef173a
22 changed files with 62 additions and 65 deletions
|
@ -36,7 +36,6 @@ CSS rules inside of a `<style>` tag are automatically scoped to that component.
|
|||
|
||||
For best results, you should only have one `<style>` tag per-Astro component. This isn’t necessarily a limitation, but it will often result in better-optimized CSS in your final build. When you're working with pages, the `<style>` tag can go nested inside of your page `<head>`. For standalone components, the `<style>` tag can go at the top-level of your template.
|
||||
|
||||
|
||||
```html
|
||||
<!-- Astro Component CSS example -->
|
||||
<style>
|
||||
|
@ -53,9 +52,13 @@ For best results, you should only have one `<style>` tag per-Astro component. Th
|
|||
<!-- Astro Page CSS example -->
|
||||
<html>
|
||||
<head>
|
||||
<style>...</style>
|
||||
<style>
|
||||
...
|
||||
</style>
|
||||
</head>
|
||||
<body>...</body>
|
||||
<body>
|
||||
...
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
|
@ -120,6 +123,7 @@ const name = "Your name here";
|
|||
<h1>Hello {name}!</h1>
|
||||
</div>
|
||||
```
|
||||
|
||||
#### Dynamic Attributes
|
||||
|
||||
```astro
|
||||
|
@ -145,7 +149,6 @@ const items = ["Dog", "Cat", "Platipus"];
|
|||
</ul>
|
||||
```
|
||||
|
||||
|
||||
### Component Props
|
||||
|
||||
An Astro component can define and accept props. Props are available on the `Astro.props` global in your frontmatter script.
|
||||
|
@ -295,7 +298,6 @@ Astro provides a `<slot />` component so that you can control where any children
|
|||
<!-- TODO: https://github.com/snowpackjs/astro/issues/360
|
||||
Document Named Slots -->
|
||||
|
||||
|
||||
## Comparing `.astro` versus `.jsx`
|
||||
|
||||
`.astro` files can end up looking very similar to `.jsx` files, but there are a few key differences. Here's a comparison between the two formats.
|
||||
|
@ -351,7 +353,4 @@ import thumbnailSrc from './thumbnail.png';
|
|||
|
||||
If you’d prefer to organize assets alongside Astro components, you may import the file in JavaScript inside the component script. This works as intended but this makes `thumbnail.png` harder to reference in other parts of your app, as its final URL isn’t easily-predictable (unlike assets in `public/*`, where the final URL is guaranteed to never change).
|
||||
|
||||
|
||||
[code-ext]: https://marketplace.visualstudio.com/items?itemName=astro-build.astro-vscode
|
||||
|
||||
|
||||
|
|
|
@ -208,4 +208,3 @@ export async function createCollection() {
|
|||
- API Reference: [collection](/reference/api-reference#collections-api)
|
||||
- API Reference: [createCollection()](/reference/api-reference#createcollection)
|
||||
- API Reference: [Creating an RSS feed](/reference/api-reference#rss-feed)
|
||||
|
||||
|
|
|
@ -51,8 +51,6 @@ Besides the obvious performance benefits of sending less JavaScript down to the
|
|||
|
||||
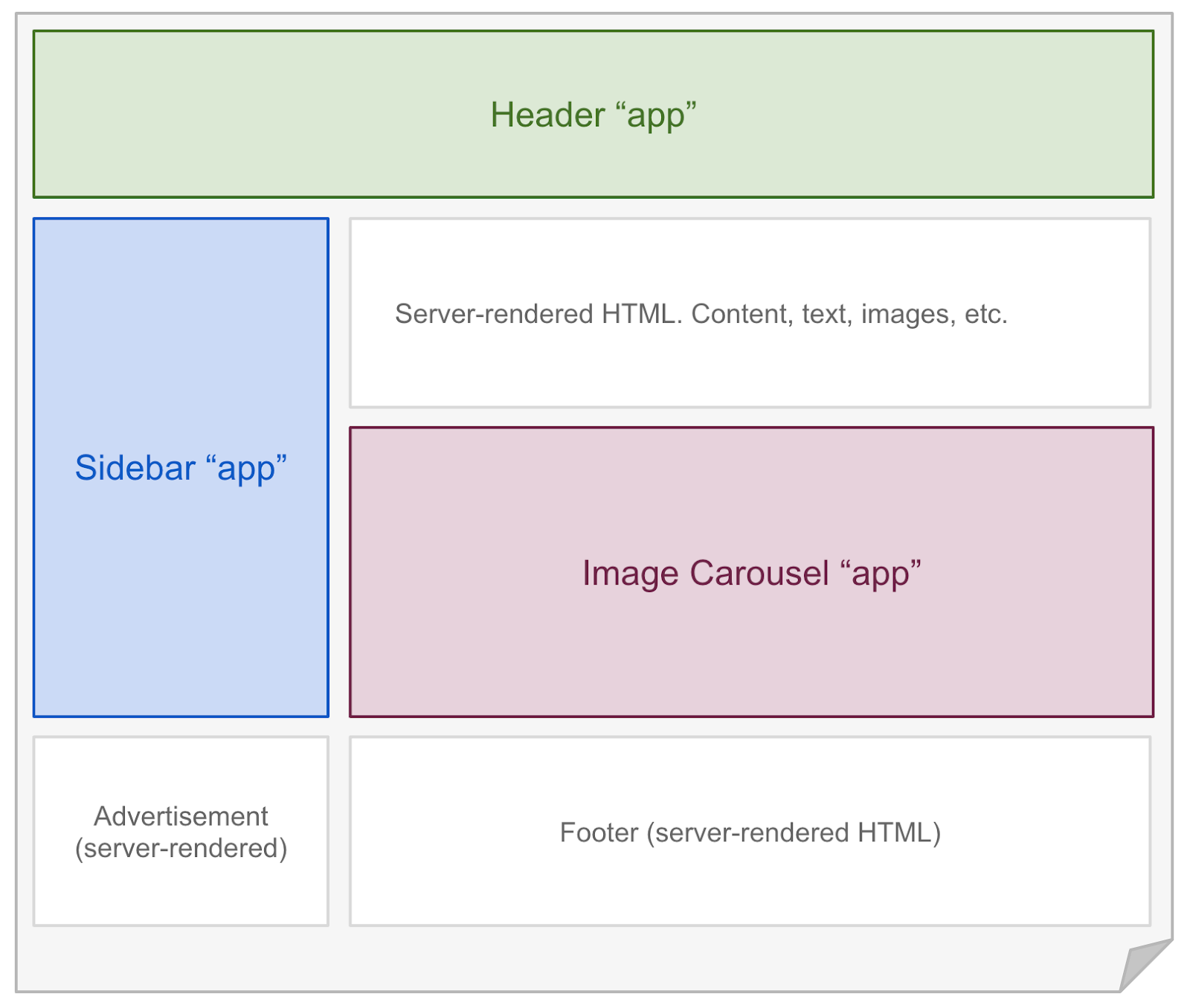
|
||||
|
||||
|
||||
|
||||
## Hydrate Interactive Components
|
||||
|
||||
Astro renders every component on the server **at build time**. To hydrate components on the client **at runtime**, you may use any of the following `client:*` directives. A directive is a component attribute (always with a `:`) which tells Astro how your component should be rendered.
|
||||
|
@ -68,15 +66,19 @@ import MyReactComponent from '../components/MyReactComponent.jsx';
|
|||
```
|
||||
|
||||
### `<MyComponent client:load />`
|
||||
|
||||
Hydrate the component on page load.
|
||||
|
||||
### `<MyComponent client:idle />`
|
||||
|
||||
Hydrate the component as soon as main thread is free (uses [requestIdleCallback()][mdn-ric]).
|
||||
|
||||
### `<MyComponent client:visible />`
|
||||
|
||||
Hydrate the component as soon as the element enters the viewport (uses [IntersectionObserver][mdn-io]). Useful for content lower down on the page.
|
||||
|
||||
### `<MyComponent client:media={QUERY} />`
|
||||
|
||||
Hydrate the component as soon as the browser matches the given media query (uses [matchMedia][mdn-mm]). Useful for sidebar toggles, or other elements that should only display on mobile or desktop devices.
|
||||
|
||||
## Can I Hydrate Astro Components?
|
||||
|
|
|
@ -54,12 +54,10 @@ import BaseLayout from '../layouts/BaseLayout.astro'
|
|||
</BaseLayout>
|
||||
```
|
||||
|
||||
|
||||
## Nesting Layouts
|
||||
|
||||
You can nest layouts when you want to create more specific page types without copy-pasting. It is common in Astro to have one generic `BaseLayout` and then many more specific layouts (`PostLayout`, `ProductLayout`, etc.) that reuse and build on top of it.
|
||||
|
||||
|
||||
```astro
|
||||
---
|
||||
// src/layouts/PostLayout.astro
|
||||
|
@ -126,6 +124,7 @@ Layouts are essential for Markdown files. Markdown files can declare a layout in
|
|||
title: Blog Post
|
||||
layout: ../layouts/PostLayout.astro
|
||||
---
|
||||
|
||||
This blog post will be **rendered** inside of the `<PostLayout />` layout.
|
||||
```
|
||||
|
||||
|
|
|
@ -10,6 +10,7 @@ Astro includes an opinionated folder layout for your project. Every Astro projec
|
|||
- `package.json` - A project manifest.
|
||||
|
||||
The easiest way to set up your new project is with `npm init astro`. Check out our [Installation Guide](/quick-start) for a walkthrough of how to set up your project automatically (with `npm init astro`) or manually.
|
||||
|
||||
## Project Structure
|
||||
|
||||
```
|
||||
|
|
|
@ -11,7 +11,6 @@ Astro pages have access to the global `fetch()` function in their setup script.
|
|||
|
||||
Even though Astro component scripts run inside of Node.js (and not in the browser) Astro provides this native API so that you can fetch data at page build time.
|
||||
|
||||
|
||||
```astro
|
||||
---
|
||||
const response = await fetch('http://example.com/movies.json');
|
||||
|
@ -22,6 +21,7 @@ console.log(data);
|
|||
<!-- Output the result to the page -->
|
||||
<div>{JSON.stringify(data)}</div>
|
||||
```
|
||||
|
||||
### Top-level await
|
||||
|
||||
`await` is another native JavaScript feature that lets you await the response of some asynchronous promise ([MDN](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/await)). Astro supports `await` in the top-level of your component script.
|
||||
|
|
|
@ -20,8 +20,6 @@ The following guides are based on some shared assumptions:
|
|||
}
|
||||
```
|
||||
|
||||
|
||||
|
||||
## Building The App
|
||||
|
||||
You may run `npm run build` command to build the app.
|
||||
|
@ -65,7 +63,8 @@ By default, the build output will be placed at `dist/`. You may deploy this `dis
|
|||
|
||||
cd -
|
||||
```
|
||||
> You can also run the above script in your CI setup to enable automatic deployment on each push.
|
||||
|
||||
> You can also run the above script in your CI setup to enable automatic deployment on each push.
|
||||
|
||||
### GitHub Actions
|
||||
|
||||
|
@ -138,8 +137,8 @@ Then, set up a new project on [Netlify](https://netlify.com) from your chosen Gi
|
|||
|
||||
If you don't want to use the `netlify.toml`, when you go to [Netlify](https://netlify.com) and set up up a new project from Git, input the following settings:
|
||||
|
||||
- **Build Command:** `astro build` or `npm run build`
|
||||
- **Publish directory:** `dist`
|
||||
- **Build Command:** `astro build` or `npm run build`
|
||||
- **Publish directory:** `dist`
|
||||
|
||||
Then hit the deploy button.
|
||||
|
||||
|
|
|
@ -29,7 +29,7 @@ export function getUser() {
|
|||
}
|
||||
|
||||
// src/index.js
|
||||
import {getUser} from './user.js';
|
||||
import { getUser } from './user.js';
|
||||
```
|
||||
|
||||
All browsers now support ESM, so Astro is able to ship this code directly to the browser during development.
|
||||
|
|
|
@ -32,7 +32,6 @@ To create global styles, add a `:global()` wrapper around a selector (the same a
|
|||
|
||||
📚 Read our full guide on [Astro component syntax](/core-concepts/astro-components#css-styles) to learn more about using the `<style>` tag.
|
||||
|
||||
|
||||
## Cross-Browser Compatibility
|
||||
|
||||
We also automatically add browser prefixes using [Autoprefixer][autoprefixer]. By default, Astro loads the [Browserslist defaults][browserslist-defaults], but you may also specify your own by placing a [Browserslist][browserslist] file in your project root.
|
||||
|
@ -57,6 +56,7 @@ All styles in Astro are automatically [**autoprefixed**](#cross-browser-compatib
|
|||
---
|
||||
|
||||
## Frameworks and Libraries
|
||||
|
||||
### 📘 React / Preact
|
||||
|
||||
`.jsx` files support both global CSS and CSS Modules. To enable the latter, use the `.module.css` extension (or `.module.scss`/`.module.sass` if using Sass).
|
||||
|
|
|
@ -90,4 +90,3 @@ Success! You're now ready to start developing! Jump over to our [Quickstart Guid
|
|||
📚 Learn more about Astro's project structure in our [Project Structure guide](/core-concepts/project-structure).
|
||||
📚 Learn more about Astro's component syntax in our [Astro Components guide](/core-concepts/astro-components).
|
||||
📚 Learn more about Astro's file-based routing in our [Routing guide](core-concepts/astro-pages).
|
||||
|
||||
|
|
|
@ -65,6 +65,7 @@ const data = Astro.fetchContent('../pages/post/*.md'); // returns an array of po
|
|||
`Astro.site` returns a `URL` made from `buildOptions.site` in your Astro config. If undefined, this will return a URL generated from `localhost`.
|
||||
|
||||
## Collections API
|
||||
|
||||
### `collection` prop
|
||||
|
||||
```jsx
|
||||
|
@ -176,4 +177,3 @@ export default function () {
|
|||
```
|
||||
|
||||
[canonical]: https://en.wikipedia.org/wiki/Canonical_link_element
|
||||
|
||||
|
|
|
@ -17,8 +17,8 @@ import { Markdown } from 'astro/components';
|
|||
```
|
||||
|
||||
See our [Markdown Guide](/guides/markdown-content) for more info.
|
||||
<!-- TODO: We should move some of the specific component info here. -->
|
||||
|
||||
<!-- TODO: We should move some of the specific component info here. -->
|
||||
|
||||
## `<Prism />`
|
||||
|
||||
|
|
|
@ -21,7 +21,6 @@ Specifies should port to run on. Defaults to `3000`.
|
|||
|
||||
Builds your site for production.
|
||||
|
||||
|
||||
## Global Flags
|
||||
|
||||
### `--config path`
|
||||
|
|
Loading…
Reference in a new issue